If you're looking for help with C#, .NET, Azure, Architecture, or would simply value an independent opinion then please get in touch here or over on Twitter.
Below is a random collection of things that have tickled me and/or I’ve greatly appreciated as I’ve started to write more code in F#.
Exceptions
I love how concise it is to declare and use an F# exception:
exception OoopsButterFingers let catchTheBall () = raise OoopsButterFingers
Or to attach some data:
exception GetOffMyLawn of string let strayOntoLawn () = raise (GetOffMyLawn "Oi!")
Single Case Discriminated Unions
Leaving the question of mutability aside I’m sure we’ve all got examples of mis-assigning IDs in models:
class User { public string UserId { get; set; } } class Order { public string OrderedByUserId { get; set; } public string OrderId { get; set; } } User user = getUser(); Order newOrder = new Order { OrderedByUserId = "newid", OrderId = user.UserId // oops, we've mis-assigned an ID };
It’s easily done right? And we could certainly improve that in C# but I love how simple this is in F#:
type UserId = | UserId of string type OrderId = | OrderId of string type User = { userId: UserId } type Order = { orderedByUserId: UserId orderId: OrderId } let user = { userId = UserId("abc") } // This won't compile - both assignments will fail with type mismatches let newOrder = { orderedByUserId = "newId" orderId = user.userId }
Currying is not named after a vindaloo
While explaining the concept of currying to a friend he asked the obvious question “why the heck is it called currying?”. I had no idea and briefly entertained the notion that it had been named in jest after, well, a curry. Which gave me a good chuckle.
However it turns out its named after an American mathematician and logician, one Haskell Curry. And so at the same time I also learned why the language Haskell is called Haskell. One of those things you feel somewhat of an ignorant philistine over not knowing.
And with that I’ll leave you with a photo of a delicious curry.
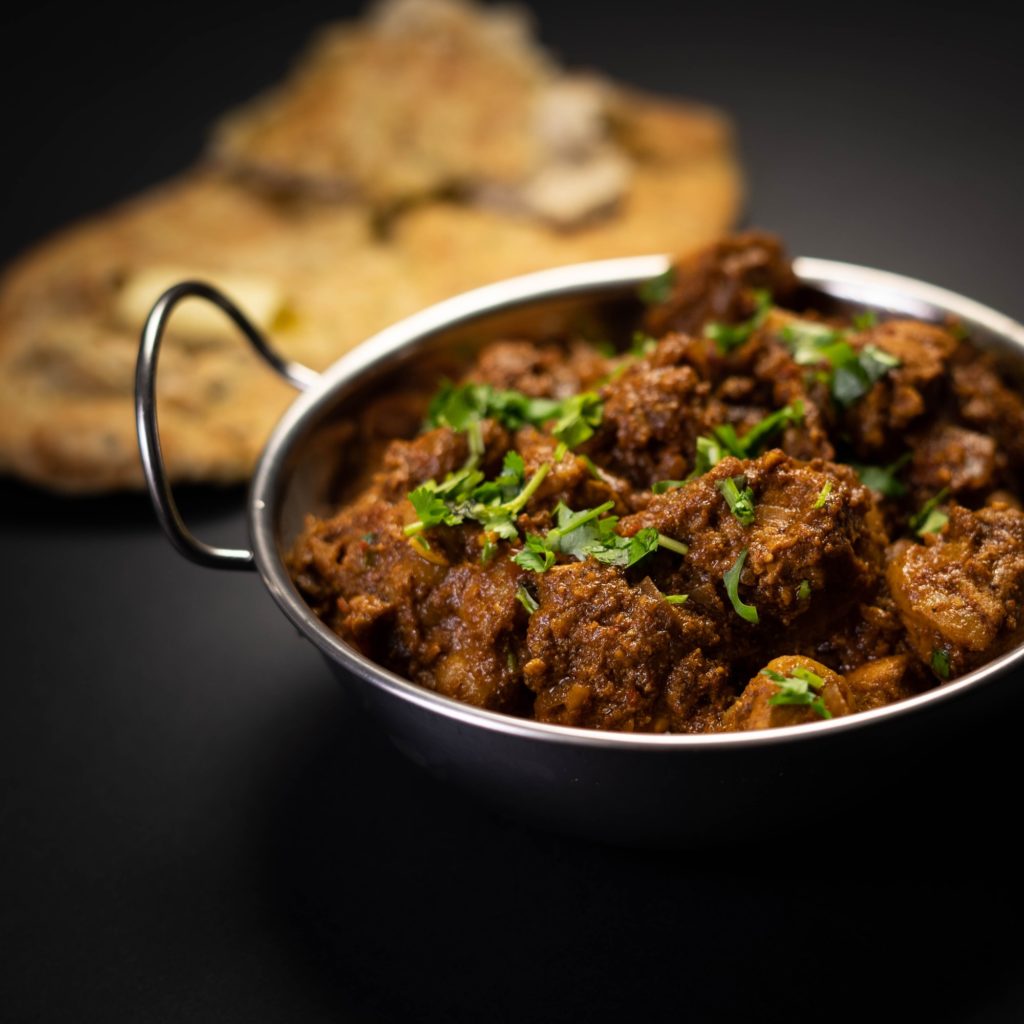
Photo via Unsplash – Steven Bennett
Recent Comments